
Findlevels Algorithm
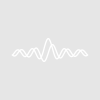
fay.tor
Tue, 08/30/2016 - 08:03 am
I use the function "Findlevels" to treat my signals and it works very well. I wondered if it was possible to have the algorithm of this function because I want to reproduce exactly my program (procedure) in another programming language, C # for example. Is it possible ?
Many thanks,
August 31, 2016 at 07:50 am - Permalink
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
August 31, 2016 at 07:56 am - Permalink
I would like to have the same code and keep the same parameters used in the function Find Levels such as "Box" and "Level".
I could not find a simple way to do it :(
September 2, 2016 at 05:57 am - Permalink
As to your ending question ... I suggest you should contact WaveMetrics directly. I imagine that releasing code or even commenting that they might release it is not something they will do on a public forum. Finally, I might suggest that at best, you will get references to literature and still have to develop your own code. In other words, I seriously doubt that you will be handed a "code package" that you can just duplicate and use directly.
Which brings me back to my starting question.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
September 3, 2016 at 07:34 am - Permalink
@jjweimer : We use Igor Pro for R&D. The goal was to test the algorithm on a large set of data then to make it an embedded software.
Since my last post here I have no longer worked on the issue. But, today I suddenly had a flash ;-).
Here is my solution
//-----------------------------------------------------------------------------------------
// LOOK FOR INDEX
//-----------------------------------------------------------------------------------------
// This function act as the Igor's function "FindLevels"
//-----------------------------------------------------------------------------------------
Function/WAVE LookForIndex(dataWave,level, boxSize)
Wave dataWave
Variable level // threshold to be crossed
Variable boxSize // box size for sliding average, should preferably be an odd number
boxSize = ceil((boxSize-1)/2) // take the half size of the box
Variable length = numpnts(dataWave)
Variable i, j
Variable slidingAverage
Variable lookForRisingEdge = 1
Make/O/N=10000 indexWave // we assume that we have less than 10000 crossing level. If not, change this value.
for(i = 0; i < length; i+=1)
slidingAverage = mean(dataWave,i-boxSize,i+boxSize) // comput the sliding average
if ( (slidingAverage > level) && (lookForRisingEdge == 1)) // look for rising edge
indexWave[j] = i
lookForRisingEdge = 0
j=j+1
elseif ( (slidingAverage) < level && (lookForRisingEdge == 0 )) // look for falling edge
indexWave[j] = i
lookForRisingEdge = 1
j=j+1
endif
endfor
Redimension /N=(j) indexWave
return indexWave // All "X" values at which the "level" was crossed
End
Here is an example (attached image) with the same parameters on a noisy data:
LookForIndex(myData,1, B=151)
FindLevels/B=151/Q/P/D=levelWave myData,1
December 2, 2016 at 08:18 am - Permalink