
Get a list of all loaded waves
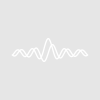
ryleighmoore
Wed, 05/20/2015 - 01:03 pm
I have been searching for a way to get a list of all loaded waves in an igor experiment for a few weeks now. I have tried WaveList("*",";","") but that gets all the waves in the folder even if they aren't already loaded. I have also looked at the waveselectorwidget example but I am still not able to get it.
Thanks for the help.
Ryleigh
When you open an Igor experiment file, all waves in the experiment are loaded into memory. So "even if they aren't already loaded" does not make sense.
WaveList("*",";","") gives you a list of all waves in the current data folder. Note that a "data folder" is not the same as a folder on disk.
To learn about data folders, execute:
DisplayHelpTopic "Data Folders"
May 20, 2015 at 02:14 pm - Permalink
STATIC CONSTANT kRECURSE =1
STATIC CONSTANT kGETPATH = 2
STATIC CONSTANT kADDCOLON = 4
STATIC CONSTANT kNOPACKAGES = 8
//Procedures for making lists of Igor objects from folders and graphs, with specializations for making the kinds of lists used in popup menus
//******************************************************************************************************
// GUIPListObjs returns a semicolon-separated list of all the objects of the slelected type (objectType)
// in the given folder (sourceFoldrStr)whose names match the given string (matchStr)
// Last Modified 2014/12/04 by Jamie Boyd
Function/s GUIPListObjs (sourceFolderStr, objectType, matchStr, options, erstring, [sepStr])
string sourceFolderStr // can be either ":" or "" to specify the current data folder. You can also use a full or partial data folder path.
variable objectType // 1 = waves, 2= numeric variables, 3= string variables, 4 = data folders
string matchstr // limit list of objects with this wildcard-enhanced string. use "*" to get all objects
variable options // bit 0=1: set to do a recursive call through all subfolders of the source folder
// bit 1 =2: set to get full or relative filepaths depending on sourceFolderStr. unset to get just the objects with no path.
// bit 2 =4: If listing folders, set to suffix folder names with a colon, good for building filepaths, unset for no colon
// bit 3 = 8: do not list packages folder or items from packages folder
string erstring // a string to return if no objects are found
string sepStr // optional separator string for returned list. Default is ";"
if (ParamIsDefault(sepStr))
sepStr = ";"
endif
// return error message if folder does not exist
// why the "\\M1(" ? It is a formatting code for disabling choices in pop-up menus
if (!(datafolderexists (sourceFolderStr)))
return "\\M1(" + sourceFolderStr + " does not exist."
endif
// make sure sourceFolderStr ends with a colon
sourceFolderStr = RemoveEnding (sourceFolderStr, ":") + ":"
// if giving path, or when doing recursive calls, prepend each object name with sourceFolder
string endStr = sepStr, prependStr = SelectString ((options & (kGETPATH + kRECURSE)), "", sourceFolderStr)
DFREF sourceDFR = $sourceFolderStr
// if listing folders, end each folder name with a colon if requested, and always add a list separator
if (objectType ==4)
endStr = SelectString ((options & kADDCOLON), sepStr, ":" + sepStr)
endif
// start return list with matching objects in sourceFolder
variable iObj
string objName, returnList = ""
// if not listing packages folder, check for it when we are looking through root folder
if (((options & kNOPACKAGES) && (cmpStr (sourceFolderStr, "root:") ==0)) && (objectType ==4))
for (iObj = 0 ; ; iObj += 1)
objName = GetIndexedObjNameDFR(sourceDFR, objectType, iObj)
if (strlen (objName) > 0)
if ((stringmatch(objname, matchStr )) && (stringMatch (objname, "!packages")))
returnList += prependStr + possiblyquoteName (objname) + endStr
endif
else
break
endif
endfor
else
for (iObj = 0 ; ; iObj += 1)
objName = GetIndexedObjNameDFR(sourceDFR, objectType, iObj)
if (strlen (objName) > 0)
if (stringmatch(objname, matchStr ))
returnList += prependStr + possiblyquoteName (objname) + endStr
endif
else
break
endif
endfor
endif
// If recursive, iterate though all folders in sourceFolder
// in recursive calls, set erString to "", so user's erStr is not added to the return list for every empty subfolder, only if all folders are empty
if (options & kRECURSE)
variable iFolder
string aFolder
if ((options & kNOPACKAGES) && (cmpStr (sourceFolderStr, "root:") ==0))
for (iFolder =0; ; iFolder +=1)
aFolder = GetIndexedObjNameDFR(sourceDFR, 4, iFolder)
if (strlen (aFolder) > 0)
if (StringMatch (aFolder, "!packages")) // if not listing packages folder, check for it when we are looking through root folder
returnList += GUIPListObjs (sourceFolderStr + possiblyquotename (aFolder), objectType, matchStr, options , "", sepStr = sepStr)
endif
else
break
endif
endfor
else
for (iFolder =0; ; iFolder +=1)
aFolder = GetIndexedObjNameDFR(sourceDFR, 4, iFolder)
if (strlen (aFolder) > 0)
returnList += GUIPListObjs (sourceFolderStr + possiblyquotename (aFolder), objectType, matchStr, options , "", sepStr = sepStr)
else
break
endif
endfor
endif
endif
// if no list was made, and this is the starting call, return user's error message
if (strlen (returnList) < 2)
return erstring
else
return returnList
endif
end
To see a list of all the waves in the experiment:
print GUIPListObjs ("root:", 1, "*", 1, "")
If you are looking for a list of files in a directory on disk, well I have a function to do that as well, from the same package. It has many options, and you have to make an Igor path.
//Returns a list of files of a particular type, whose names match the match string, and are located in the disk directory pointed to by the given path
// Last modified: 2014/12/04 by Jamie Boyd
// Added option to follow items that are shortcuts
// Mod 2014/10/22 by Jamie Boyd: Includes option to also list creation date and modification date
Function/S GUIPListFiles (ImportpathStr, fileTypeOrExtStr, matchStr, options, erstring, [sepStr])
String ImportPathStr // Name of an Igor Path
String fileTypeOrExtStr // macintosh file type (4 characters) or Windows extension, e.g., ".txt", or "dirs" to list directories
String MatchStr // string to match file names for listing. Wildcard enabled. Pass "*" to list all files
variable options // bit 0=1: set to do a recursive call through all directories of the source directory
// bit 1 =2: set to get full file paths. unset to get just the object names with no path.
// bit 2 =4: set to strip three character file name extensions from returned file names
// bit 3 = 8: set to allow user to set ImportPath if it does not already exist.
// bit 4 = 16: set to list file creation dates after each file name
// bit 5 = 32: set to list file modification dates after each file name, or after each creation date
// bit 6 = 64: set to list alias/shortcuts that point to files, and to list contents of aliases to folders-must be listing full paths
string erstring // a string to return if no objects are found
string sepStr // optional separator string for returned list. Default is ";"
if (ParamIsDefault(sepStr))
sepStr = ";"
endif
// we have to look for shorcuts/aliases differently on Mac vs PC
string fileAliasExt = ".lnk", fldrAliasExt = ".lnk"
if (cmpStr ( IgorInfo (2), "Macintosh") ==0)
fileAliasExt = "alis"
fldrAliasExt ="fdrp"
endif
// if the Igor path does not exist, let the user set it, if that option is chosen
string sourceDirStr
PathInfo $ImportPathStr
if (!(V_Flag))
if (options & 8)
NewPath /O/M= "Set Path to FIles of type " + fileTypeOrExtStr $ImportPathStr
if (V_flag)
return "\\M1(Invalid Path"
else
PathInfo $ImportPathStr
sourceDirStr = S_path
endif
else
return "\\M1(Invalid Path"
endif
else
sourceDirStr = S_path
endif
// if giving path, prepend each object name with sourceFolder
string prependStr = SelectString ((options & kGETPATH), "", sourceDirStr)
// string prependStr = SelectString (((options & kGETPATH) || (options & 64)), "", sourceDirStr)
// iterate through files in this directory
string afileName, allFiles, returnList = "" // lists of files that we will return
variable iFile, nFiles, nameLen, aliasPathDepth
if (cmpStr (fileTypeOrExtStr, "dirs") ==0) // looking for directories
AllFiles = IndexedDir($ImportpathStr, -1, (options & 4))
nFiles = itemsinlist (AllFiles, ";")
Make/N=(nFiles)/T/FREE tempFiles = StringFromList(p, AllFiles)
for (iFile =0; iFile < nFiles; iFile +=1)
afileName =tempFiles [iFile]
if (stringmatch(afileName, matchStr))
returnList += prependStr + aFileName + sepStr
if (options & 48)
GetFileFolderInfo /P=$ImportpathStr/Q afileName
if (options & 16)
returnList += secs2Date (V_creationDate, -2, "/") + "/" + Secs2Time(V_creationDate, 2) + sepStr
endif
if (options & 32)
returnList += secs2Date (V_modificationDate, -2, "/") + "/" + Secs2Time(V_creationDate, 2) + sepStr
endif
endif
endif
endfor
if (options & 64)
// now look for aliases to folders, probably fewer of these, so not bothering making a temp wave
AllFiles = IndexedFile ($ImportPathStr, -1, fldrAliasExt)
for (iFile =0, nFiles = itemsinlist (AllFiles, ";"); iFile < nFiles; iFile +=1)
afileName = StringFromList (iFile, allFiles, ";")
GetFileFolderInfo /P=$ImportpathStr /Q aFilename
if ((V_isAliasShortcut) && (cmpStr (S_aliasPath [Strlen (s_aliasPath) -1], ":") ==0))
aliasPathDepth = itemsinlist (s_aliasPath, ":") -1
afileName = StringFromList(aliasPathDepth, S_aliasPath, ":" )
if (stringmatch(afileName, matchStr))
returnList +=S_aliasPath + sepStr
if (options & 48)
GetFileFolderInfo S_aliasPath
if (options & 16)
returnList += secs2Date (V_creationDate, -2, "/") + "/" + Secs2Time(V_creationDate, 2) + sepStr
endif
if (options & 32)
returnList += secs2Date (V_modificationDate, -2, "/") + "/" + Secs2Time(V_creationDate, 2) + sepStr
endif
endif
endif
endif
endfor
endif
else //looking for files
AllFIles = IndexedFile ($ImportPathStr, -1, fileTypeOrExtStr)
nFiles = itemsinlist (AllFiles, ";")
Make/O/N=(nFiles)/T/FREE tempFiles = StringFromList(p, AllFiles)
for (iFile =0; iFile < nFiles; iFile +=1)
afileName =tempFiles [iFile]
if (stringmatch(afileName, matchStr))
if (options & 4)
NameLen = strlen (afileName)
if ((cmpstr (afileName [NameLen - 4], ".")) == 0)
returnList += prependStr + aFileName [0, NameLen - 5] + sepStr
else
returnList += prependStr + aFileName + sepStr
endif
else
returnList += prependStr + aFileName + sepStr
endif
if (options & 48)
GetFileFolderInfo /P=$ImportpathStr/Q afileName
if (options & 16)
returnList += secs2Date (V_creationDate, -2, "/") + "/" + Secs2Time(V_creationDate, 2) + sepStr
endif
if (options & 32)
returnList += secs2Date (V_modificationDate, -2, "/") + "/" + Secs2Time(V_creationDate, 2) + sepStr
endif
endif
endif
endfor
if (options & 64)
// now look for aliases to files, probably fewer of these, so not bothering making a temp wave
AllFiles = IndexedFile ($ImportPathStr, -1, fileAliasExt)
for (iFile =0, nFiles = itemsinlist (AllFiles, ";"); iFile < nFiles; iFile +=1)
afileName = StringFromList (iFile, allFiles, ";")
GetFileFolderInfo /P=$ImportpathStr /Q aFilename
if ((V_isAliasShortcut) && (cmpStr (S_aliasPath [Strlen (s_aliasPath) -1], ":") !=0))
aliasPathDepth = itemsinlist (s_aliasPath, ":") -1
afileName = StringFromList(aliasPathDepth, S_aliasPath, ":" )
if (stringmatch(afileName, matchStr))
returnList +=S_aliasPath + sepStr
if (options & 48)
GetFileFolderInfo S_aliasPath
if (options & 16)
returnList += secs2Date (V_creationDate, -2, "/") + "/" + Secs2Time(V_creationDate, 2) + sepStr
endif
if (options & 32)
returnList += secs2Date (V_modificationDate, -2, "/") + "/" + Secs2Time(V_creationDate, 2) + sepStr
endif
endif
endif
endif
endfor
endif
endif
// If recursive, iterate though all folders in sourceFolder
// in recursive calls, set erString to "", so user's erStr is not added to the return list for every empty subfolder, only if all folders are empty
if (options & kRECURSE)
string subFolders
variable iFolder, nFolders
if ((options & kGETPATH) || (options & 64)) // if getting full path, just list the files
subFolders = IndexedDir($ImportpathStr, -1, 1)
nFolders = itemsinList (subFolders, ";")
for (iFolder =0; iFolder < nFolders; iFolder +=1)
NewPath /O/Q GuipListRecPath, stringFromList (iFolder, subFolders, ";")
returnList += GUIPListFiles ("GuipListRecPath", fileTypeOrExtStr, matchStr, options, "", sepStr = sepStr)
endfor
if (options & 64)
AllFiles = IndexedFile ($ImportPathStr, -1, fldrAliasExt)
for (iFile =0, nFiles = itemsinlist (AllFiles, ";"); iFile < nFiles; iFile +=1)
afileName = StringFromList (iFile, allFiles, ";")
GetFileFolderInfo /P=$ImportpathStr /Q aFilename
if ((V_isAliasShortcut) && (cmpStr (S_aliasPath [Strlen (s_aliasPath) -1], ":") ==0))
NewPath /O/Q GuipListRecPath, S_aliasPath
returnList += GUIPListFiles ("GuipListRecPath", fileTypeOrExtStr, matchStr, options, "", sepStr = sepStr)
endif
endfor
endif
else // if not getting full path, need to make relative path from starting folder
string dirStr = stringFromList (itemsinList (sourceDirStr, ":")-1, sourceDirStr, ":") + ":"
string subList
string subDirStr
subFolders = IndexedDir($ImportpathStr, -1, 0)
nFolders = itemsinList (subFolders, ";")
for (iFolder =0; iFolder < nFolders; iFolder +=1)
subDirStr = stringFromList (iFolder, subFolders, ";")
NewPath /O/Q GuipListRecPath, sourceDirStr + subDirStr
subList = GUIPListFiles ("GuipListRecPath", fileTypeOrExtStr, matchStr, options, "", sepStr = sepStr)
for (iFile=0, nFiles = itemsinList (subList, sepStr); iFile < nFiles; iFile +=1)
returnList += subDirStr + ":" + stringFromList (iFile, subList, sepStr) + sepStr
if ((options & 16) || (options & 32))
iFIle +=1
returnList += stringFromList (iFile, subList, sepStr) + sepStr
endif
if ((options & 16) && (options & 32))
iFIle +=1
returnList += stringFromList (iFile, subList, sepStr) + sepStr
endif
endfor
endfor
endif
endif
// if no list was made, and this is the starting call, return user's error message
if (strlen (returnList) < 2)
return erString
else
return returnList
endif
end
For example, the following command:
print GUIPListFiles ("MyPath", "????", "*", 8, "")
will make a new Igor Path named MyPath and the user will have an opportunity to choose the folder the path points to. It will return a list of files of all file types.
cheers,
Dr. Jamie Boyd, Ph.D.
May 20, 2015 at 10:51 pm - Permalink
May 21, 2015 at 01:25 pm - Permalink