
Flip rows in 3d matrix
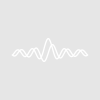
bs
Thu, 01/29/2015 - 02:08 pm
Sadly, the best solution I've come up with so far involves way too many
imagetransform
s and is way too slow.1)
Duplicate
the matrix (already off to a bad start)2) Delete all even columns from one matrix and the odds from the other: iterate
imagetransform removeyplane
3) on the odd matrix: iterate
imagetransform flipcols
(could rotate the matrix to avoid iterating and speed up a bit)4) iterate
imagetransform getplane
on the odds and imagetransform insertplane
on the evensThere has to be a more efficient way to do this. Any ideas?
If it helps:
The data is acquired as a one dimension wave with (1024*1024*200) rows and I redimension it to (1024,1024,200).
wave0 = p // populate with some data - this takes a long time
redimension/N=(1024, 1024, 200) wave0
make/O/N=(1024, 1024, 200) wave1
wave1[][][] = (q & 1) ? wave0[1023 - p][q][r] : wave0[p][q][r] // reverse odd columns
The last step took about 30 seconds on my rather old PC. I don't know how this timing compares with other methods?
HTH,
Kurt
January 30, 2015 at 12:02 am - Permalink
This should set only odd columns starting from column 1.
See the discussion starting with "You can specify not only a range but also a point number increment" here:
DisplayHelpTopic "Indexing and Subranges"
January 30, 2015 at 02:29 am - Permalink
That is clever!
A quick test shows this is about 3 times faster than the ?: statement.
January 30, 2015 at 03:15 am - Permalink
1. Use WaveTransform with the keyword flip. The result is then stored in W_flipped.
2. Create two waves of dimensions (1024*1024*200) say w1 and w2.
3. Set w1 to 1 for odd cols points and 0 otherwise.
w2=mod(q,2)==1 ? 0:1
4. Set w2 to 1 for even cols points and 0 otherwise.
5. Execute
HTH,
A.G.
WaveMetrics, Inc.
January 30, 2015 at 07:36 am - Permalink
Thanks a lot.
Perhaps I misunderstood something but it seems that
WaveTransform flip
isn't giving the expected rotation of the 3d volume.
I replaced it with:
imagetransform flipplanes m_flipped
matrixop/o m_flipped2 = transposeVol(m_flipped, 3)
February 2, 2015 at 03:16 am - Permalink