
Inconsistent type for a wave reference - complex wave and extract statement
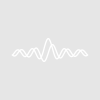
Henry S.
Thu, 11/06/2014 - 03:47 pm
I'm working with complex waves. Depending on conditions, I'd like to either simply define complex wave cw or extract it from another complex wave. Here's a simplified example:
function freewavecollision(a)
variable a
switch (a)
case 1:
make/free/c/n=1 wc = { cmplx(1,1) }
break
default:
make/free/c/n=3 longwc = { cmplx(0,0), cmplx(5,5), cmplx (10,10) }
extract/free longwc, wc, p==1
endswitch
print wc
end
variable a
switch (a)
case 1:
make/free/c/n=1 wc = { cmplx(1,1) }
break
default:
make/free/c/n=3 longwc = { cmplx(0,0), cmplx(5,5), cmplx (10,10) }
extract/free longwc, wc, p==1
endswitch
print wc
end
When I try to compile this code, I get a dialog box with "Inconsistent type for a wave reference". When I dismiss the dialog box,
wc
in extract/free longwc, wc, p==1
is highlighted. I've tried adding /c/o
to the extract statement to no avail. I also tried this with real numbers and wc is assigned successfully.I've coded in a work-around, but would like to get the logic above to work.
I'm using 32-bit Igor 6.3.5.5 in Windows 8.0.
Thank you!
Henry
variable a
make/o/c/free/n=1 wc
switch (a)
case 1:
wc = { cmplx(1,1) }
break
default:
make/free/c/n=3 longwc = { cmplx(0,0), cmplx(5,5), cmplx (10,10) }
wc = longwc[1]
endswitch
print wc
end
'_free_'[0]= {cmplx(1,1)}
•freewavecollision(6)
'_free_'[0]= {cmplx(5,5)}
November 10, 2014 at 08:09 am - Permalink
As you suggested, it's possible to work around the compiler error by not using
extract
and redimensioning the output wave (wc) as necessary. Instead, I used a couple of temporary waves:variable a
switch (a)
case 1:
make/free/c/n=1 wc1 = { cmplx(1,1) }
wave wc = wc1
break
default:
make/free/c/n=3 longwc = { cmplx(0,0), cmplx(5,5), cmplx (10,10) }
extract/free longwc, wc2, p==1
wave wc = wc2
endswitch
print wc
end
I do believe that the compiler is flagging my original code erroneously. If the equivalent is done with real numbers, it works as expected. If you really want to see something weird, while I was testing the behavior with real numbers, I accidentally mixed real and complex outputs for wave w. The code compiles and behaves as I would expect:
variable a
switch (a)
case 1:
make/free/n=1 w = { 1 }
break
default:
make/free/c/n=3 longw = { cmplx(0,0), cmplx(5,5), cmplx (10,10) }
extract/free longw, w, p==1
endswitch
print w
end
The results:
'_free_'[0]= {1}
•wavecollision(2)
'_free_'[0]= {cmplx(5,5)}
Thanks again for your suggestion. I do appreciate the helpful spirit of this community!
November 20, 2014 at 08:57 pm - Permalink
make/c a
make/c b
extract a, b, 1
end
November 21, 2014 at 12:45 am - Permalink
At least I would have guessed that
Make/O/D a
Make/O/N=0 b
Extract a, b, 1
print wavetype(b)
End
complains that b already exists. Or why is there a /O flag if it always overwrites?
November 21, 2014 at 04:31 am - Permalink
As it happens (but incorrectly documented) the overwrite pertains to the destination overwriting the source (i.e., Extract a,a,1). I'll create a ticket to have the docs changed.
Larry Hutchinson
WaveMetrics
support@WaveMetrics.com
November 21, 2014 at 10:21 am - Permalink
The OPs question still remains unsolved for me though.
November 24, 2014 at 02:14 pm - Permalink
When working with complex waves it is useful to remember that MatrixOP provides additional flexibility in that it is not fussy about complex vs real waves. In this case I'd replace the OP line:
with:
A.G.
WaveMetrics, Inc.
November 24, 2014 at 05:36 pm - Permalink
Looking back at the Extract code, I see that there is no support for a complex destination wave reference variable.
I'll look into fixing that.
At present you would have to cheat a bit. Nasty but this works:
variable a
switch (a)
case 1:
make/free/c/n=1 wc = { cmplx(1,1) }
break
default:
make/free/c/n=3 longwc = { cmplx(0,0), cmplx(5,5), cmplx (10,10) }
WAVE longwcreal= longwc
extract/free longwcreal, wcr, p==1
Redimension/C/E=1 wcr
WAVE/C wc= wcr
endswitch
print wc
end
Larry Hutchinson
WaveMetrics
support@WaveMetrics.com
November 25, 2014 at 02:48 pm - Permalink
November 26, 2014 at 02:41 pm - Permalink
This fix will be in the next overnight build. You can get that here
http://www.wavemetrics.net/Downloads/latest/
The current build date is Wed, 26 Nov, so anything after that will have the change.
Larry Hutchinson
WaveMetrics
support@WaveMetrics.com
November 27, 2014 at 07:54 am - Permalink
December 2, 2014 at 12:01 pm - Permalink