
Novice programming syntax help please (unknown/inappropriate Name or symbol)
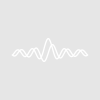
phonon
Mon, 08/05/2013 - 04:37 pm
Can anyone help me with something that must be pretty simple but it's giving me a headache. I'm guess it's just a syntax error.
In my code I've got a wave with temperatures and another couple of huge "reference" waves, one with temperature and one with a value that corresponds to each temperature.
The value is the "error" associated with that temperature reading.
I just want to compare each temperature in the small wave with all the temperatures in the huge wave then get the corresponding error value for that temperature, then add it to a smaller wave.
So far I've got this little nested loop thing.
Wavestats TCerror //V_npnts = num pts in TCerror wave
npts_TCerror = V_npnts
Wavestats AbsTemp //V_npnts = num pts in AbsTemp wave
npts_AbsTemp = V_npnts
for (i = 0; i < npts_TCerror; i+=1)
for (j = 0; j < npts_AbsTemp; j+=1)
if (Temp[i] == AbsTemp[j])
deltatwt[i] = SigmaDT[j]
endif
endfor
endfor
npts_TCerror = V_npnts
Wavestats AbsTemp //V_npnts = num pts in AbsTemp wave
npts_AbsTemp = V_npnts
for (i = 0; i < npts_TCerror; i+=1)
for (j = 0; j < npts_AbsTemp; j+=1)
if (Temp[i] == AbsTemp[j])
deltatwt[i] = SigmaDT[j]
endif
endfor
endfor
Well, the compiler absolutely does not like anything I put here:
if (Temp[i] == //XXXX//)
What am I doing wrong? I've done these if statements before.
August 5, 2013 at 06:14 pm - Permalink
August 6, 2013 at 05:49 am - Permalink
I do. That's just a snippet.
No matter WHAT I put there, I get an error.
I'll keep trying.
edit:
Ok, it was fine with Temp for some reason, but not fine with the other thing there. Earlier I Make those waves and then directly after use them in this if statement. I guess I need to make them or load them, then make wave variables for them to use them?
August 6, 2013 at 10:03 am - Permalink
Can the interp() function do cubic spline instead of linear with a flag?
August 6, 2013 at 09:53 am - Permalink
If you will post a complete function that we can try to compile then we will be able to diagnose what the problem is.
If necessary create a simplified version of your actual function that shows the error.
August 6, 2013 at 10:02 am - Permalink
Not to my knowledge, take a look at the interpolate XOP
EDIT: Apparently Interpolate2 with the /t=2 flag (default) does cubic splines.
August 6, 2013 at 10:08 am - Permalink
I just edited the earlier post. I think it's just the fact that I thought that if you make a wave or load a wave in a procedure then you can just use it without making a variable.
Incomplete code below:
String listoftempwaves = WaveList("KRDGC*", ";", "")
String listofdtwaves = Wavelist("Delta*",";","")
String listofhtrpwrwaves = Wavelist ("PWR*",";","")
String listofampswaves = Wavelist ("Amps*",";","")
String listofvoltswaves = Wavelist ("Volts*",";","")
Variable i
Variable j
Variable nsets = ItemsInList(listoftempwaves)
Variable pwr
Variable tcon
Variable tcerr
Make/N=(nsets) Temp,TempSD,TCon,TCerr
Variable refNum
Open /R /MULT=0 /M="Select the Weight (StDev) File" refNum
LoadWave/A/D/H/J/O/W/K=1/L={0,1,0,0,0} S_fileName //This will load waves AbsTemp and SigmaDT
Wave deltawt
Wavestats SigmaDT
Variable npts_SigmaDT = V_npnts
for (i = 0; i < nsets; i += 1)
Wave tempwave = $StringFromList(i, listoftempwaves)
Wave deltawave = $StringFromList(i, listofdtwaves)
Wave pwrwave = $StringFromList(i, listofhtrpwrwaves)
Wave ampswave = $StringFromList(i, listofampswaves)
Wave voltswave = $StringFromList(i, listofvoltswaves)
Wavestats tempwave
Temp[i] = V_avg
TempSD[i] = V_sdev
for (j = 0; j < npts_SigmaDT; j+=1)
if (Temp[i] == AbsTemp[j])
deltatwt[i] = SigmaDT[j]
endif
endfor
Wavestats ampswave
for (j = 0;j < V_npnts; j+= 1)
Wave ampserr
Wave voltserr
Wave pwrwt
htrpwr = ampswave[j]*voltswave[j]
ampserr[j] = ampswave[j]*6.6e-4+20e-6
voltserr[j] = voltswave[j]*1.5e-4+1.5e-3
htrpwrwt[j] = (sqrt((AmpsErr[j]/ampswave[j])^2+(VoltsErr[j]/voltswave[j])^2))*pwr
endfor
Curvefit/ODR=2 line pwrwave/X=deltatwave /I=1 /W=deltatwt /XW=pwrwt
endfor
End
August 6, 2013 at 10:13 am - Permalink
Cool. This just might save me lots of time. I would still have to load waves from a file though, either a pre-interpolated one or one that will be interpolated every time the function runs.
August 6, 2013 at 10:14 am - Permalink
wave TempSD
wave TCon
wave TCerr
August 6, 2013 at 10:56 am - Permalink
Variable tcerr
Next add this after the LoadWave statement:
Next the wave name here does not agree with the Wave statement above:
Then you've got some inconsistencies between wave declarations and wave assignment statements in the for loop.
In some cases Igor automatically creates wave references but not after LoadWave. Carefully read the following:
including the following subtopic "Automatic Creation of Wave References"
August 6, 2013 at 11:19 am - Permalink
Oh right. I think those were leftovers.
Oh good, you can do a list like this. That'll save some space.
Thanks a lot.
Now I get the way wave references work.
Sorry for being so clueless.
August 6, 2013 at 11:48 am - Permalink