
Extracting a value from a text wave
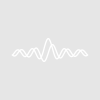
puglie12
Thu, 07/04/2013 - 08:28 am
I have a text wave containing a variety of weather conditions (i.e. cloudy, mostly cloudy, clear, rainy, etc.). I want to assign values for each condition such that I can add up how many "cloudy" days there were in a certain month. So I need, for example, to write a function that looks for "cloudy" in my weather wave and creates a new wave where this value now is 1, then looks for "mostly cloudy" and assigns a value of 2, etc. This way, I can add up all of the days that had "1" and all of the days that had "2" and figure out how many days were cloudy, mostly cloudy, etc.
I am having trouble extracting these values from a text wave. Can you please help?
Thank you,
Stephanie
To understand it I recommend reading from the bottom (high level function) to the top (low level function).
The CountCondition1 and CountCondition2 functions are two ways to do the same thing. For small wave sizes it does not matter which you use. For large wave sizes I suspect CountCondition1 would be faster but I would have to test to be sure.
Make /T /N=0 /O conditions
conditions[0] = {"cloudy"} // Append a condition
conditions[1] = {"sunny"} // Append another condition
conditions[2] = {"sunny"}
conditions[3] = {"cloudy"}
conditions[4] = {"rainy"}
End
// This is one way to do it using the Extract operation.
Function CountCondition1(w, condition)
Wave/T w // Text wave containing conditions
String condition // "sunny", "cloudy", or "rainy"
// Create free wave containing points set to the specified condition.
// The free wave is automatically killed when the function returns.
Extract /FREE w, temp, CmpStr(w[p],condition)==0
Variable result = numpnts(temp)
return result
End
// This is another way to do it using a loop. Either way is fine.
Function CountCondition2(w, condition)
Wave/T w // Text wave containing conditions
String condition // "sunny", "cloudy", or "rainy"
Variable result = 0
Variable numPoints = numpnts(w)
Variable i
for(i=0; i<numPoints; i+=1)
String str = w[i]
if (CmpStr(str,condition) == 0)
result +=1
endif
endfor
return result
End
Function Demo()
Setup()
Wave/T conditions // Created by Setup
Variable numSunny = CountCondition2(conditions, "sunny")
Variable numCloudy = CountCondition2(conditions, "cloudy")
Variable numRainy = CountCondition2(conditions, "rainy")
Printf "Sunny: %d; Cloudy: %d; Rainy: %d;\r", numSunny, numCloudy, numRainy
End
July 4, 2013 at 11:30 am - Permalink
July 4, 2013 at 01:43 pm - Permalink