
COnverting from bytes to a float
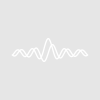
amankjain
Tue, 03/19/2013 - 09:17 pm
I am trying to convert a portion of the response from a serial port that is 4 of the bytes into a single precision floating point.
I am reading the response into a string as
string returnStr
VDTRead2 returnStr
//Creating a wave of unsigned bytes as follows
Make/B/U/N=4 waveToRead
//Assigning wave from the response
waveToRead[0] = char2Num(returnStr[3])
waveToRead[1] = char2Num(returnStr[4])
waveToRead[2] = char2Num(returnStr[5])
waveToRead[3] = char2Num(returnStr[6])
//cast wave to single point float
Redimension/S waveToRead
Am I on the right path?
Thanks
AJ
If that doesn't work try reversing the order of the bytes.
However it seems that you should be using VDTReadBinary2 to read the 4 bytes directly into a variable. Since you reading 6 bytes and only the last four are of interest you can use VDTReadBinary2 to discard two bytes. Putting it altogether you would get something like this:
VDTReadBinary2 /TYPE=(0x14) junk // Read junk as 16-bit unsigned
VDTReadBinary2 /TYPE=2 value // Read value as single-precision float
If your data is little-endian then you need to add /B to flip the byte order.
March 20, 2013 at 05:52 am - Permalink
VDTRead2 returnStr
substr = returnStr[3,6]
//the following assumes that substr is a multiple of 4 bytes, you can convert as many numbers as you want.
//a wavetype of 2 represents single precision float. Use /E to perform endian conversion.
sockitstringtowave/DEST=waveToRead 2, returnStr
March 20, 2013 at 06:12 am - Permalink