
Get the mean value for a row of a 2D wave
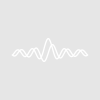
finn
Fri, 10/07/2011 - 07:55 am
with the following function I am able to get the mean value of a defined region in a column (vertical dimension):
wave1(0)=mean(2Dwave,10,1)
Can anybody tell me how to get the mean value of a defined region in a row (horizontal dimension) of a 2D wave?
cheers,
Finn
Wave wave2D
Variable rn //row number
Variable x1,x2
Duplicate /O wave2D Rwave //row wave
Redimension /N=(1,-1) Rwave //redimension to a single row
Rwave = wave2D[rn][q] //assign the r column
MatrixOp /O Rwave = Rwave^t //transpose row into a column
return mean(Rwave, x1,x2)
end
October 7, 2011 at 09:01 am - Permalink
Make/O/N=(5,3) mat = p + 10*q
Edit mat
MatrixOp rowSums = SumRows(mat)
AppendToTable rowSums
End
October 7, 2011 at 10:06 am - Permalink
I prefer a slightly simpler version:
Wave wave2D
Variable rn //row number
Variable x1,x2
MatrixOP/O/Free w=row(wave2D,rn)^t
return mean(w, x1,x2)
End
A.G.
WaveMetrics, Inc.
October 7, 2011 at 01:50 pm - Permalink
thanks very much! With your help it is working now.
cheers,
Finn
October 8, 2011 at 03:56 am - Permalink
wave w;
variable n;
make/n=0/free wc;
if(n < dimsize(w,0))
matrixop/free wc = row(w,n);
endif
return wc;
end
function/wave col(w,n)
wave w;
variable n;
make/n=0/free wc;
if(n < dimsize(w,1))
matrixop/free wc = col(w,n);
endif
return wc;
end
These functions are cool because you can pass them right into a another function. So if I wanted to find the mean of values 3 to 20 in row 15 of some 2D wave, w, I can run this:
I keep loads of utility functions like these in Ipfs that load automatically with Igor at startup so they are always there for me to use.
November 3, 2011 at 11:56 pm - Permalink
Often times I want to do something like this but for all cols or rows:
Wave wave0
Variable x1
Variable x2
Make/D/O/N = (Dimsize(wave0,1)) ones = 0
ones[x1, x2] = 1
MatrixOp/O mean_rows = (wave0 x ones)/ (x2-x1+1)
End
if you do not want subranges it is even easier:
Wave wave0
Make/D/O/N = (Dimsize(wave0,1)) ones = 1
MatrixOp/O mean_rows = (wave0 x ones)/ numcols(wave0)
Make/D/O/N = (Dimsize(wave0,0)) ones = 1
MatrixOp/O mean_cols = (wave0^t x ones)/numrows(wave0)
Killwaves ones
End
Just be careful - in this simple example it does not account for Nans or Infs
November 4, 2011 at 08:35 am - Permalink
November 8, 2011 at 12:16 pm - Permalink