
converting byte waves to floating point numbers
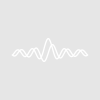
harneit
Wed, 02/09/2011 - 08:35 pm
Anyway, does anyone have an easier way than type-casting via structures like so:
structure dbyte_struct
char bytes[8]
endstructure
structure dval_struct
double value
endstructure
function BytesToDouble(byteWave, byteOrder)
wave byteWave
variable byteOrder
struct dbyte_struct db
struct dval_struct dv
variable k
for( k = 0; k < 8; k += 1 )
db.bytes[k] = byteWave[k]
endfor
string temp
StructPut/S db, temp
StructGet/S/B=(byteOrder) dv, temp
return dv.value
end
char bytes[8]
endstructure
structure dval_struct
double value
endstructure
function BytesToDouble(byteWave, byteOrder)
wave byteWave
variable byteOrder
struct dbyte_struct db
struct dval_struct dv
variable k
for( k = 0; k < 8; k += 1 )
db.bytes[k] = byteWave[k]
endfor
string temp
StructPut/S db, temp
StructGet/S/B=(byteOrder) dv, temp
return dv.value
end
I've had to solve similar problems.
Check out the Sockit XOP.
Use SOCKITwavetoString to convert the bytes into a string, then use SOCKITstringtowave to put the bytes into the wavetype of your choice. It will do big endian conversions if you ask it to. It's all done in memory with memcpy.
Alternatively, you should be able to use redimension with the /E flag. That's probably safer if you're distributing code.
Andy
February 9, 2011 at 09:53 pm - Permalink
Redimension
operation actually does the entire job. So all I have to do isRedimension /D /E=1 bw
where
bw
is a byte wave made byMake/B/U bw
as in my previous code. If the byte order is in doubt, I can then useRedimension /D /E=2 bw
to swap the byte order back and forth...
This is brilliant. The documentation nearly hides this cool feature, the only information I could find in the help files was
Uncharacteristically terse...although spot-on in hindsight.
Thanks for pointing that out, Andy!
February 10, 2011 at 02:39 am - Permalink