
Using wave masks for wave indexing?
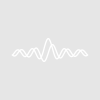
saalvarez
Fri, 07/01/2011 - 02:58 am
I recently started using Igor pro (and programming in general), so please forgive me if my explanation is not clear.
I am trying to make an igor pro version of a matlab code I have to stitch images together. I work at a beamline where we collect scattering data from a detector. The detector plate has 5 gaps (horizontal lines) where we loose scattering data. To go around this, we move the detector up a few mm (30pixels) to make up for the data we loose. These are the two images I want to stitch. This is the approach the code I am trying to translate to igor pro uses (maybe the wave indexing on igor pro can give me a more efficient way to do this...)
1) I start with two matrices, A and B , of the same dimensions (981X1043)
2) I make 6 waves of (1200x1200), lets call them IMG_UP,IMG_DOWN,t1,t2,t3,Test
3) I copy A to IMG_DOWN. I copy B, which is the image after moving 30 pixles up, to IMG_UP, but I shift the columns by 30 pixels (same amount I physically moved the detector. This is with the intention of adding the two matrices)
4) Now, I want to determine where I have the gaps on each image ( 0 intensity points) and where I have data (non-zero points). To do this, I use logical expressions like the following to obtain masks in terms of zeros and 1's:
t1= ( IMG_DOWN == 0 )
t2= ( IMG_UP == 0 )
t3= ( C != 0 & D != 0 )
From t1 and t2 I obtain wave masks of the gaps on the detector( zero intensity values). From t3 I obtain the values where I have data points.
Now I want to use the images A and B, and the masks (t1,t2,t3), to compose the stitched image on the wave "Test". This is where I need help. In matlab, you can write something like this:
Test(t1)=IMG_UP(t1)
Here t1 contains a mask for the values that are zero on IMAGE_DOWN, and I want to use this mask to look for those values in IMAGE_UP and copy them on Test, which will be my final stitched image. The code continues with two more lines using the same kind of indexing and then I redimension my matrix to the original size. This works fine in matlab, but I would like to know if there is an equivalent way to do this in igor pro. I tried something like:
Test(t1[p][q])=IMG_UP(t1[p][q])
in igor pro on the cmd window. I don't get errors from doing this, but it is not transferring any values (wave test is still zero after doing these operations). I can probably find in the images the rows/columns where I have the gaps, use wave indexing to select the data points I want and make the stitched image, but I found this to be useful to know and would appreciate feedback. The question is, can I use a wave for wave indexing?
Thanks in advance, and again, my apologies if the problem was not stated properly (this is literally my first time writing code of any sort and in addition to that, english is not my first language =])
Best,
Steven
ALS- Lawrence Berkeley National Lab.
Wave img_down,img_up
Variable counter // declare variable for the 'for'-loop
for (counter = 0; counter < DimSize(img_down,1); counter += 1) // go through cols; number of cols from img_down is the limiter (dimsize)
if (img_down[0][counter] == 0) // condition: if first point is zero in current col (I guess all points are zero then)
img_down[][counter] = img_up[p][counter-30] // insert data 30 cols before current col (this substitutes manual shifting) from img_up
endif
endfor
End
Paste this code into the procedure window (ctrl+m) and run from the command line: combine(your wave A here, your wave B here).
I counldn't test this. So if it doesn't work, upload a test experiment with data to play around with. I assume by shifting wave b by 30 pixels, you get the data line up 30 columns earlier. That's why I introduced this counter-30 stuff. Could be the other way around. I also don't know why you need 1200x1200 matrices with the data being smaller in every direction. Hope it works for you.
July 1, 2011 at 05:26 am - Permalink
I think you might benefit from using MatrixOP and possibly ImageTransform and ImageBlend. There are a few stages in this process that I find questionable so feel free to write to me directly if you want to look at the whole process.
Now for the specific question:
You can create a mask in MatrixOP using an expression of the form:
MatrixOP/O mask1=greater(inWave,0)
This will set a mask to 1 where inWave is greater than zero. Similarly,
MatrixOP/O mask2=equal(inWave,0)
will set to 1 all pixels for which inWave is zero.
The above are far more efficient than your expressions for t1, t2, t3.
Since it appears that you are trying to insert the images of smaller dimensions into images of larger dimensions I think you will find that an efficient way to do so involves:
Redimension/N=(1200,1200) WaveA
ImageTransform/IOFF={dx,dy,0} WaveA
I am not sure what is meant by:
Test(t1)=IMG_UP(t1)
This could possibly be handled by MatrixOP using waveMap() or some other means that depend on your application.
A.G.
WaveMetrics, Inc.
July 1, 2011 at 12:08 pm - Permalink
Thanks to both of you for your prompt responses. I am really excited to be learning how to program in Igor and I am happy to know that there is such a good supporting community here. =]
I asked for some help at work and my problem was on the indexing. This is how my indexing looks now:
t01= (gm00 == 0);
t02= (gm30 == 0);
t03= (gm00 !=0 & gm30 != 0);
test = t02 == 1 ? gm00[p][q] : test
test = t01 == 1 ? gm30[p][q]: test
test = t03 !=0 ?( gm00[p][q]+gm00[p][q])/2 : test
I also reduced the size of my matrices and just added the 30 extra rows that I needed. I was literally just trying to use the same procedure I had in matlab, but I didn't realize that bigger matrices= unnecessary higher memory load. I am looking into both of your suggestions to see how I can improve my code. Again, thanks for the help! It is much appreciated
July 5, 2011 at 12:40 am - Permalink