
Trouble with time wave
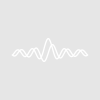
chemgoof
Wed, 03/04/2015 - 03:09 pm
The .txt time format is in am/pm (ex. 4:00 AM 4:01 AM... 4:00 PM). When I load using my function, it loads the times as the same regardless of am or pm. So 4:00 AM on 9/24 is seen the same as 4:00 PM (both loading as 04:00:00). I have attached a sample .txt and the code is below. The function is meant to load an entire folder so the .txt needs to be in its own folder to load. I have also sent this to the wavemetrics support. Any help is appreciated!
#pragma rtGlobals=3 // Use modern global access method and strict wave access.
#pragma rtGlobals=3 // Use modern global access method and strict wave access.
Menu "Weather"
"Load Weather Data...", LoadWeatherFiles("")
End
Function LoadWeatherFile(pathName, fileName, makeTable)
String pathName // Name of an Igor symbolic path or "".
String fileName // Name of file or full path to file.
Variable makeTable // 1 to make table, 0 to not make table
// First get a valid reference to a file.
if ((strlen(pathName)==0) || (strlen(fileName)==0))
// Display dialog looking for file.
Variable refNum
Open/D/R/F="*.txt"/P=$pathName refNum as fileName
fileName = S_fileName // S_fileName is set by Open/D
if (strlen(fileName) == 0) // User cancelled?
return -1
endif
endif
// Now load the data. The /V flag specifies the accepted delimiters in the data file.
// Add the /A flag if you don't want the "Loading Delimited Text" dialog.
// Add the /O flag if you want to overwrite existing waves with the same names.
// "Date" and "Time" are not available as wave names because they are Igor function names
String columnInfoStr = " "
columnInfoStr += "N='MET_TimeW',T=4;"
columnInfoStr += "N='TimeW',F=7;"
columnInfoStr += "N='Temp_C';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='Hum_Per';"
columnInfoStr += "N='Dew_Pt_C';"
columnInfoStr += "N='Wind_Speed';"
columnInfoStr += "N='Wind_Dir',F=-2;"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='Wind_Chill_C';"
columnInfoStr += "N='Heat_Index_C';"
columnInfoStr += "N='THW_Index_C';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='Press_Bar';"
columnInfoStr += "N='Precip_Inch';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
String delimiters = "\t," // Tab and comma-delimited
String skipChars = "\" " // Skip quotes around date and time and spaces before commas
LoadWave /J /L={0,2,0,0,0}/D/N /K=0 /V={delimiters,skipChars,0,0} /B=columnInfoStr /R={English, 1, 2, 1, 1, "Month/DayofMonth/Year", 40} /P=$pathName fileName
Variable numWavesLoaded = V_flag // V_flag is set by LoadWave
if (numWavesLoaded != 12)
Print "Error loading file - wrong number of waves loaded"
return -1
endif
// Create reference to waves created by LoadWave
Wave MET_TimeW,TimeW
MET_timew += TimeW // Add time to date to obtain date/time
KillWaves/Z TimeW // This is no longer needed
return 0 // Success
End
Function ConcatenateWeatherFile(pathName, fileName)
String pathName
String fileName
// Create a new data folder
NewDataFolder/O/S WeatherTemp
Variable result = LoadWeatherFile(pathName, fileName, 0)
SetDataFolder :: // Back to original data folder
if (result != 0)
return result // Error loading new waves
endif
// Concatenate new waves onto old
Concatenate/NP {:WeatherTemp:MET_TimeW},MET_TimeW
Concatenate/NP {:WeatherTemp:Temp_C},Temp_C
Concatenate/NP {:WeatherTemp:Hum_Per},Hum_Per
Concatenate/NP {:WeatherTemp:Dew_Pt_C},Dew_Pt_C
Concatenate/NP {:WeatherTemp:Wind_Speed},Wind_Speed
Concatenate/NP {:WeatherTemp:Wind_Dir},Wind_Dir
Concatenate/NP {:WeatherTemp:Wind_Chill_C},Wind_Chill_C
Concatenate/NP {:WeatherTemp:Heat_Index_C},Heat_Index_C
Concatenate/NP {:WeatherTemp:THW_Index_C},THW_Index_C
Concatenate/NP {:WeatherTemp:Press_Bar},Press_Bar
Concatenate/NP {:WeatherTemp:Precip_Inch},Precip_Inch
// Kill temp data folder
KillDataFolder/Z :WeatherTemp
return 0 // Success
End
Function LoadWeatherFiles(pathName)
String pathName // Name of symbolic path or "" to get dialog
String fileName
Variable index=0
if (strlen(pathName)==0) // If no path specified, create one
NewPath/O temporaryPath // This will put up a dialog
if (V_flag != 0)
return -1 // User cancelled
endif
pathName = "temporaryPath"
endif
Variable result
do // Loop through each file in folder
fileName = IndexedFile($pathName, index, ".txt")
if (strlen(fileName) == 0) // No more files?
break // Break out of loop
endif
result = ConcatenateWeatherFile(pathName, fileName)
if (result == 0) // Did LoadAndGraph succeed?
// Print the graph.
fileName = WinName(0, 1) // Get the name of the top graph
//String cmd
//sprintf cmd, "PrintGraphs %s", fileName
//Execute cmd // Explained below.
//DoWindow/K $fileName // Kill the graph
//KillWaves/A/Z // Kill all unused waves
endif
index += 1
while (1)
if (Exists("temporaryPath")) // Kill temp path if it exists
KillPath temporaryPath
endif
Wave MET_TimeW,TimeW,Temp_C,Hum_Per,Dew_Pt_C,Wind_Speed,Wind_Dir,Wind_Chill_C,Heat_Index_C,THW_Index_C,Press_Bar,Precip_Inch
Edit MET_TimeW,Temp_C,Hum_Per,Dew_Pt_C,Wind_Speed,Wind_Dir,Wind_Chill_C,Heat_Index_C,THW_Index_C,Press_Bar,Precip_Inch
ModifyTable format(MET_TimeW)=8, width(MET_TimeW)=150
Sort MET_TimeW Temp_C,Hum_Per,Dew_Pt_C,Wind_Speed,Wind_Dir;DelayUpdate
Sort MET_TimeW Wind_Chill_C,Heat_Index_C,THW_Index_C,Press_Bar,Precip_Inch
Sort MET_TimeW MET_TimeW
DoWindow/K Weather
DoWindow/C/T Weather,"Weather Data"
return 0 // Signifies success.
End
#pragma rtGlobals=3 // Use modern global access method and strict wave access.
Menu "Weather"
"Load Weather Data...", LoadWeatherFiles("")
End
Function LoadWeatherFile(pathName, fileName, makeTable)
String pathName // Name of an Igor symbolic path or "".
String fileName // Name of file or full path to file.
Variable makeTable // 1 to make table, 0 to not make table
// First get a valid reference to a file.
if ((strlen(pathName)==0) || (strlen(fileName)==0))
// Display dialog looking for file.
Variable refNum
Open/D/R/F="*.txt"/P=$pathName refNum as fileName
fileName = S_fileName // S_fileName is set by Open/D
if (strlen(fileName) == 0) // User cancelled?
return -1
endif
endif
// Now load the data. The /V flag specifies the accepted delimiters in the data file.
// Add the /A flag if you don't want the "Loading Delimited Text" dialog.
// Add the /O flag if you want to overwrite existing waves with the same names.
// "Date" and "Time" are not available as wave names because they are Igor function names
String columnInfoStr = " "
columnInfoStr += "N='MET_TimeW',T=4;"
columnInfoStr += "N='TimeW',F=7;"
columnInfoStr += "N='Temp_C';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='Hum_Per';"
columnInfoStr += "N='Dew_Pt_C';"
columnInfoStr += "N='Wind_Speed';"
columnInfoStr += "N='Wind_Dir',F=-2;"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='Wind_Chill_C';"
columnInfoStr += "N='Heat_Index_C';"
columnInfoStr += "N='THW_Index_C';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='Press_Bar';"
columnInfoStr += "N='Precip_Inch';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
String delimiters = "\t," // Tab and comma-delimited
String skipChars = "\" " // Skip quotes around date and time and spaces before commas
LoadWave /J /L={0,2,0,0,0}/D/N /K=0 /V={delimiters,skipChars,0,0} /B=columnInfoStr /R={English, 1, 2, 1, 1, "Month/DayofMonth/Year", 40} /P=$pathName fileName
Variable numWavesLoaded = V_flag // V_flag is set by LoadWave
if (numWavesLoaded != 12)
Print "Error loading file - wrong number of waves loaded"
return -1
endif
// Create reference to waves created by LoadWave
Wave MET_TimeW,TimeW
MET_timew += TimeW // Add time to date to obtain date/time
KillWaves/Z TimeW // This is no longer needed
return 0 // Success
End
Function ConcatenateWeatherFile(pathName, fileName)
String pathName
String fileName
// Create a new data folder
NewDataFolder/O/S WeatherTemp
Variable result = LoadWeatherFile(pathName, fileName, 0)
SetDataFolder :: // Back to original data folder
if (result != 0)
return result // Error loading new waves
endif
// Concatenate new waves onto old
Concatenate/NP {:WeatherTemp:MET_TimeW},MET_TimeW
Concatenate/NP {:WeatherTemp:Temp_C},Temp_C
Concatenate/NP {:WeatherTemp:Hum_Per},Hum_Per
Concatenate/NP {:WeatherTemp:Dew_Pt_C},Dew_Pt_C
Concatenate/NP {:WeatherTemp:Wind_Speed},Wind_Speed
Concatenate/NP {:WeatherTemp:Wind_Dir},Wind_Dir
Concatenate/NP {:WeatherTemp:Wind_Chill_C},Wind_Chill_C
Concatenate/NP {:WeatherTemp:Heat_Index_C},Heat_Index_C
Concatenate/NP {:WeatherTemp:THW_Index_C},THW_Index_C
Concatenate/NP {:WeatherTemp:Press_Bar},Press_Bar
Concatenate/NP {:WeatherTemp:Precip_Inch},Precip_Inch
// Kill temp data folder
KillDataFolder/Z :WeatherTemp
return 0 // Success
End
Function LoadWeatherFiles(pathName)
String pathName // Name of symbolic path or "" to get dialog
String fileName
Variable index=0
if (strlen(pathName)==0) // If no path specified, create one
NewPath/O temporaryPath // This will put up a dialog
if (V_flag != 0)
return -1 // User cancelled
endif
pathName = "temporaryPath"
endif
Variable result
do // Loop through each file in folder
fileName = IndexedFile($pathName, index, ".txt")
if (strlen(fileName) == 0) // No more files?
break // Break out of loop
endif
result = ConcatenateWeatherFile(pathName, fileName)
if (result == 0) // Did LoadAndGraph succeed?
// Print the graph.
fileName = WinName(0, 1) // Get the name of the top graph
//String cmd
//sprintf cmd, "PrintGraphs %s", fileName
//Execute cmd // Explained below.
//DoWindow/K $fileName // Kill the graph
//KillWaves/A/Z // Kill all unused waves
endif
index += 1
while (1)
if (Exists("temporaryPath")) // Kill temp path if it exists
KillPath temporaryPath
endif
Wave MET_TimeW,TimeW,Temp_C,Hum_Per,Dew_Pt_C,Wind_Speed,Wind_Dir,Wind_Chill_C,Heat_Index_C,THW_Index_C,Press_Bar,Precip_Inch
Edit MET_TimeW,Temp_C,Hum_Per,Dew_Pt_C,Wind_Speed,Wind_Dir,Wind_Chill_C,Heat_Index_C,THW_Index_C,Press_Bar,Precip_Inch
ModifyTable format(MET_TimeW)=8, width(MET_TimeW)=150
Sort MET_TimeW Temp_C,Hum_Per,Dew_Pt_C,Wind_Speed,Wind_Dir;DelayUpdate
Sort MET_TimeW Wind_Chill_C,Heat_Index_C,THW_Index_C,Press_Bar,Precip_Inch
Sort MET_TimeW MET_TimeW
DoWindow/K Weather
DoWindow/C/T Weather,"Weather Data"
return 0 // Signifies success.
End
March 4, 2015 at 03:10 pm - Permalink
Except that the time is NOT actually in am/pm format, it is in a/p format:
Date Time Out Temp Temp Hum Pt. Speed Dir Run Speed Dir Chill Index Index Index Bar Rain Rate Rad. Energy Rad. Index Dose UV D-D D-D Temp Hum Dew Heat EMC Density ET Samp Tx Recept Int. 10/02/13 11:56 p 8.2 8.2 8.2 97 7.8 0.4 SE 0.03 0.4 ESE 8.2 8.4 8.4 --- 1023.5 0.00 0.0 --- --- --- --- --- --- 0.007 0.000 13.9 73 9.2 13.6 14.18 1.2285 0.00 23 1 100.0 1
The second column has "11:56 p".
This isn't a format recognized by LoadWave. You may need to load the file using FReadLine and sscanf.
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
March 4, 2015 at 05:02 pm - Permalink
Menu "Weather"
"Load Weather Data...", LoadWeatherFiles("")
End
// HR:
// AMPMTimeAdjustment(theTime, flag)
// Returns the necessary adjustment in seconds to convert a time from AM/PM representation
// to 24 hour representation.
Function AMPMTimeAdjustment(theTime, flag)
Variable theTime
String flag // Must be either "a" or "p"
Variable adjustment = 0
Variable secondsIn12Hours = 43200
if (CmpStr(flag, "a") == 0)
// AM: If the hour is 12:xx, subtract seconds in 12 hours
if (theTime >= secondsIn12Hours)
adjustment = -secondsIn12Hours
endif
else
// PM: Adds 12 hours unless hour is 12:xx.
if (theTime < secondsIn12Hours)
adjustment = secondsIn12Hours
endif
endif
return adjustment
End
Function LoadWeatherFile(pathName, fileName, makeTable)
String pathName // Name of an Igor symbolic path or "".
String fileName // Name of file or full path to file.
Variable makeTable // 1 to make table, 0 to not make table
// First get a valid reference to a file.
if ((strlen(pathName)==0) || (strlen(fileName)==0))
// Display dialog looking for file.
Variable refNum
Open/D/R/F="*.txt"/P=$pathName refNum as fileName
fileName = S_fileName // S_fileName is set by Open/D
if (strlen(fileName) == 0) // User cancelled?
return -1
endif
endif
// Now load the data. The /V flag specifies the accepted delimiters in the data file.
// Add the /A flag if you don't want the "Loading Delimited Text" dialog.
// Add the /O flag if you want to overwrite existing waves with the same names.
// "Date" and "Time" are not available as wave names because they are Igor function names
String columnInfoStr = " "
columnInfoStr += "N='MET_TimeW',T=4;"
columnInfoStr += "N='TimeW',F=7;"
columnInfoStr += "N='AMPM',F=-2;" // HR: Load "a" or "p" after time into text wave
columnInfoStr += "N='Temp_C';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='Hum_Per';"
columnInfoStr += "N='Dew_Pt_C';"
columnInfoStr += "N='Wind_Speed';"
columnInfoStr += "N='Wind_Dir',F=-2;"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='Wind_Chill_C';"
columnInfoStr += "N='Heat_Index_C';"
columnInfoStr += "N='THW_Index_C';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='Press_Bar';"
columnInfoStr += "N='Precip_Inch';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
columnInfoStr += "N='_skip_';"
String delimiters = "\t," // Tab and comma-delimited
delimiters += " " // HR: Add space - needed to load "a" and "p" into text wave
String skipChars = "\" " // Skip quotes around date and time and spaces before commas
LoadWave /J /L={0,2,0,0,0}/D/N /K=0 /V={delimiters,skipChars,0,0} /B=columnInfoStr /R={English, 1, 2, 1, 1, "Month/DayofMonth/Year", 40} /P=$pathName fileName
Variable numWavesLoaded = V_flag // V_flag is set by LoadWave
if (numWavesLoaded != 13) // HR: 13 because of AMPM wave
Print "Error loading file - wrong number of waves loaded"
return -1
endif
// Create reference to waves created by LoadWave
Wave MET_TimeW,TimeW
// HR: Account for "a" for AM, "p" for PM
// Add 12 hours where we have "p" unless hour is 12:xx
// Subtract 12 hours where we have "a" if hour is 12:xx
Wave/T AMPM
TimeW += AMPMTimeAdjustment(TimeW, AMPM)
KillWaves/Z AMPM // This is no longer needed
MET_timew += TimeW // Add time to date to obtain date/time
KillWaves/Z TimeW // This is no longer needed
return 0 // Success
End
Function ConcatenateWeatherFile(pathName, fileName)
String pathName
String fileName
// Create a new data folder
NewDataFolder/O/S WeatherTemp
Variable result = LoadWeatherFile(pathName, fileName, 0)
SetDataFolder :: // Back to original data folder
if (result != 0)
return result // Error loading new waves
endif
// Concatenate new waves onto old
Concatenate/NP {:WeatherTemp:MET_TimeW},MET_TimeW
Concatenate/NP {:WeatherTemp:Temp_C},Temp_C
Concatenate/NP {:WeatherTemp:Hum_Per},Hum_Per
Concatenate/NP {:WeatherTemp:Dew_Pt_C},Dew_Pt_C
Concatenate/NP {:WeatherTemp:Wind_Speed},Wind_Speed
Concatenate/NP {:WeatherTemp:Wind_Dir},Wind_Dir
Concatenate/NP {:WeatherTemp:Wind_Chill_C},Wind_Chill_C
Concatenate/NP {:WeatherTemp:Heat_Index_C},Heat_Index_C
Concatenate/NP {:WeatherTemp:THW_Index_C},THW_Index_C
Concatenate/NP {:WeatherTemp:Press_Bar},Press_Bar
Concatenate/NP {:WeatherTemp:Precip_Inch},Precip_Inch
// Kill temp data folder
KillDataFolder/Z :WeatherTemp
return 0 // Success
End
Function LoadWeatherFiles(pathName)
String pathName // Name of symbolic path or "" to get dialog
String fileName
Variable index=0
if (strlen(pathName)==0) // If no path specified, create one
NewPath/O temporaryPath // This will put up a dialog
if (V_flag != 0)
return -1 // User cancelled
endif
pathName = "temporaryPath"
endif
Variable result
do // Loop through each file in folder
fileName = IndexedFile($pathName, index, ".txt")
if (strlen(fileName) == 0) // No more files?
break // Break out of loop
endif
result = ConcatenateWeatherFile(pathName, fileName)
if (result == 0) // Did LoadAndGraph succeed?
// Print the graph.
fileName = WinName(0, 1) // Get the name of the top graph
//String cmd
//sprintf cmd, "PrintGraphs %s", fileName
//Execute cmd // Explained below.
//DoWindow/K $fileName // Kill the graph
//KillWaves/A/Z // Kill all unused waves
endif
index += 1
while (1)
if (Exists("temporaryPath")) // Kill temp path if it exists
KillPath temporaryPath
endif
Wave MET_TimeW,TimeW,Temp_C,Hum_Per,Dew_Pt_C,Wind_Speed,Wind_Dir,Wind_Chill_C,Heat_Index_C,THW_Index_C,Press_Bar,Precip_Inch
Edit MET_TimeW,Temp_C,Hum_Per,Dew_Pt_C,Wind_Speed,Wind_Dir,Wind_Chill_C,Heat_Index_C,THW_Index_C,Press_Bar,Precip_Inch
ModifyTable format(MET_TimeW)=8, width(MET_TimeW)=150
Sort MET_TimeW Temp_C,Hum_Per,Dew_Pt_C,Wind_Speed,Wind_Dir;DelayUpdate
Sort MET_TimeW Wind_Chill_C,Heat_Index_C,THW_Index_C,Press_Bar,Precip_Inch
Sort MET_TimeW MET_TimeW
DoWindow/K Weather
DoWindow/C/T Weather,"Weather Data"
return 0 // Signifies success.
End
March 4, 2015 at 05:17 pm - Permalink
March 4, 2015 at 05:22 pm - Permalink