
indexing matrices
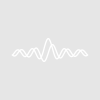
Raphael_Santos
Mon, 07/04/2016 - 08:22 pm
I'm not very experienced in programming and kind of stuck right now.
I am trying to implement the following loop on matrix calculations.
All matrices are 3x3
//R[0]= intial condition matrix
//I =identity matrix
//B= matrix
//k1,k2 = scalars
For (i=1;i<100;i+=1)
//calculate k1,k2
// calculate B
R[i]=R[i -1] x ( I + k1*B + k2*B^2)
///do some calculations using R[i]
// delete R[i-1]
endfor
//I =identity matrix
//B= matrix
//k1,k2 = scalars
For (i=1;i<100;i+=1)
//calculate k1,k2
// calculate B
R[i]=R[i -1] x ( I + k1*B + k2*B^2)
///do some calculations using R[i]
// delete R[i-1]
endfor
Could somebody help?
WAVE
statement. The scalars need to be declared as variables. It's also not a good idea to call the Identity matrix I. As you can see, i has a special meaning and Igor is case insensitive. The loop that you've written goes some way beyond your data. The number in square brackets refers to row number in your matrix, I'm guessing you need to do this on multiple matrices? The expression in the loop also won't work, because igor needs to know exactly which points it needs to work with. For your matrix calculations you are better doingMatrixOp
.July 4, 2016 at 09:52 pm - Permalink
Wave matR, matB
Variable k1, k2
Variable i
For (i = 1; i < 100; i += 1)
//calculate k1, k2
//calculate matB
MatrixOp matR = matR x (Identity(3) + k1 * matB + k2 * matB x matB)
// do some calculations using matR
endfor
end
If matB is not specified initially and matB will not be used in other procedure,
Wave matR
Variable k1, k2
Make /N=(3, 3) /O matB
Variable i
For (i = 1; i < 100; i += 1)
//calculate k1, k2
//calculate matB
MatrixOp matR = matR x (Identity(3) + k1 * matB + k2 * matB x matB)
// do some calculations using matR
endfor
KillWaves /Z matB
end
July 4, 2016 at 10:22 pm - Permalink
dear SJR51,
Thank for trying to help. The above is not an extent of my code, but more a sketch of want I want to accomplish. I know how to do the declarations, and I understand the matrix indexing. Basically, I believe my struggle resumes to this:
1. how to index a matrix as a whole inside a loop.
2. Matrixop does not seem to handle indexing. how can I use indexing inside a Matrixop operation?
Thanks
July 4, 2016 at 11:23 pm - Permalink
Dear Kanekaka
That solved my problem!
I thought I couldn't use the destination matrix on both sides of the argument of Matrixop, but this is only the case for 3d waves.
Thank you!
July 5, 2016 at 12:20 am - Permalink