
Igor newbie needs a small bit of help
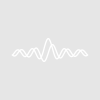
tim2020
Fri, 07/26/2013 - 06:05 am
I am new to IGOR coding and was hoping a generous member could help me code a small script.
This would help me tremendously get started with my project.
I have 10 waves (named 1 thru 10) in a folder called exp1 The organization of the folders is: root/6_12_13/animal1/exp1
I want to make a function that does the same operation to each wave in this folder.
In this case, subtract the first value in a wave from the entire wave and then divide these numbers by the initial first value. or (wave1 - first value wave1) / first value wave1
Ideally the function would create new waves and keep the original waves.
Any help would be so much appreciated. Thanks!
I think the best course of action here would be to use the WaveSelectorWidget in a panel to allow the user to browse/select a wave to process. This gives you access to the data folder path of interest, which is necessary to ensure the routine works on the data in the appropriate folder without require the user to first set the active data folder.
The WaveList function will allow you to work on any number of waves (not limited to 10) in a for loop.
July 26, 2013 at 06:37 am - Permalink
You choose all waves you want to apply the function to in the DataBrowser. Now I assume that you want to subtract/divide each wave from/by itself, right?
Then try to use some code like this:
String dummy, ActWaveList="", ActWaveNames="", ActWaveName
Variable pos, i, NumItems
//creates a wave list from the browser selection
do
dummy=GetBrowserSelection(i)
if (strlen(dummy)>0)
ActWaveList+=dummy+";"
pos=strsearch(dummy, ":",Inf,1) //separates folders and wave
ActWaveNames+=dummy[pos+1,strlen(dummy)-1]+";"
i+=1
else
NumItems=i
break
endif
while (1)
//does the mathematical operation
//the folder that contains the wave(s) has to be activated
for(i=0;i<NumItems;i+=1)
ActWaveName=StringFromList(i,ActWaveNames)
Wave ActWave=$ActWaveName //gives IGOR each wave stored in WaveList
String ActWave_op=ActWaveName+"_op"+num2str(i) //name of the new wave
Duplicate/O ActWave, $ActWave_op //new wave with the name 'ActWaveName+"_op"+num2str(i)' is generated in the data folder
Wave OpWave=$ActWave_op //gives IGOR the wave
OpWave[]=(ActWave[p]-ActWave[1])/ActWave[1]
endfor
End
Hope this helps!
Marty
July 26, 2013 at 09:25 am - Permalink
Wave w
w += 1
End
Function AddOneToWavesInList(list)
String list
Variable numItems = ItemsInList(list)
Variable i
for(i=0; i<numItems; i+=1)
String name = StringFromList(i, list)
Wave w = $name
AddOneToWave(w)
endfor
End
Function Setup()
Make/O/N=10 wave0=p, wave1=p+1, wave2=p+2
Edit wave0, wave1, wave2
End
Function Demo()
String list = WaveList("*", ";", "") // list = "wave0;wave1;wave2;"
AddOneToWavesInList(list)
End
Execute Setup() once from the command line. Then execute Demo().
July 26, 2013 at 10:57 am - Permalink