
Igor equivalent for numpy.repeat ?
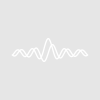
J-E Petit
Mon, 01/04/2016 - 02:59 am
and Happy New Year everyone.
Is there an inbuilt Igor equivalent for the repeat function of numpy?
for an array x=[1,2,3,4], numpy.repeat(x,4) will give [1,1,1,1,2,2,2,2,3,3,3,3,4,4,4,4]
thanks for your help!!
Cheers
J-E
Make/O/N=4 data={1, 2, 3, 4}
Rep(data, 4)
End
Function Rep(wData, vRep)
wave wData
variable vRep
variable vLenData = DimSize(wData, 0)
Make/O/N=(vLenData * vRep) wRepData
wRepData[] = wData[floor(p / vRep)]
End
Hope this helps,
Kurt
January 4, 2016 at 03:34 am - Permalink
I'm also trying to figure out how to tile a wave, but cannot find something that works properly... (something that would give [1,2,3,4,1,2,3,4,1,2,3,4,1,2,3,4] from [1,2,3,4]). A loop with Concatenate would work, but any idea on avoiding loops?
January 4, 2016 at 05:19 am - Permalink
Make/O/N=4 data={1, 2, 3, 4}
Rep(data, 4)
Rep2(data, 4)
End
Function Rep(wData, vRep)
wave wData
variable vRep
variable vLenData = DimSize(wData, 0)
Make/O/N=(vLenData * vRep) wRepData
wRepData[] = wData[floor(p / vRep)]
End
Function Rep2(wData, vRep)
wave wData
variable vRep
variable vLenData = DimSize(wData, 0)
Make/O/N=(vLenData * vRep) wRepData2
wRepData2[] = wData[mod(p, vRep)]
End
January 4, 2016 at 05:29 am - Permalink
You can use:
•make/n=16 repeatWave = xwave[floor(p/4)]
•print xwave
xwave[0]= {1,2,3,4}
•print repeatWave
repeatWave[0]= {1,1,1,1,2,2,2,2,3,3,3,3,4,4,4,4}
Try this:
•Make/N=16 dest = data[mod(p, 4)]
•print data
data[0]= {1,2,3,4}
•print dest
dest[0]= {1,2,3,4,1,2,3,4,1,2,3,4,1,2,3,4}
If you haven't already, I recommend you read the following help topic:
January 4, 2016 at 06:42 am - Permalink
Matrixop/o aa=rowrepeat(ddd,5)
redimension/n=25 aa
January 4, 2016 at 09:25 am - Permalink
January 6, 2016 at 04:04 am - Permalink