
How does overriding constant outside of main procedure file work?
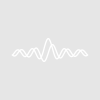
JellyGem
Wed, 11/13/2013 - 03:16 pm
I occasionally use constants in procedure files for setting certain values that I need to modify only every once in a while.
This way I can decrease the number of magic-numbers, global variables and arguments of my user functions, without losing an easy way to change certain numbers in my code, which is nice.
Recently, I have been writing a module that I will include in other procedure files and now I am trying to figure out how to use constants in them.
On one hand, I would like to write the module in such a way that, once it is complete, I don't have to modify it at all.
On the other hand, there are some numbers that I would like to change when I use it for different purposes.
To satisfy both, overriding the constant in the file which includes the module seems like a good idea, however this is not necessarily recommended in the igor pro manual.
The manual also does not give a detailed description of which non-main procedure can override the constants in which procedure.
It only says that it is decided by the order of compilation of procedure files, but doesn't say which non-main procedure becomes compiled first.
I actually tried simple tests to see how the override of constants work when I include a procedure from another non-main procedure, and under certain conditions it does work.
Is overriding a constant (or a strConstant or a function if anybody is interested) really a bad idea to do outside of the main procedure file?
And how does igor decide which procedure becomes compiled first?
variable stp
variable etp
string sname
ENDSTRUCTURE
Function Initialize(myc)
STRUCT myconstants &myc
myc.stp = 0
myc.etp = 8.314
myc.sname = "Hello World!"
return 0
end
Function DoSomething()
STRUCT myconstants myc
Initialize(myc)
print myc.sname
return 0
end
You can even store the structure and recall it later. I use this in many of my independent modules with no problem.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
November 13, 2013 at 05:14 pm - Permalink
One addition to jjweimer's approach would be to have in each procedure file a static function
InitializeStruct
which holds all settings/constants you might want to change. And then you can set the struct in each procedure file to your expected values.Alternatively you can also have a look at optional function parameters
Function doStuff([param])
variable param
if(ParamIsDefault(param))
param = paramDefault
endif
End
// default usage of doStuff
doStuff()
// special use case
doStuff(param=2)
November 14, 2013 at 12:57 am - Permalink
Thank you for your comments.
Using structs sounds like a fantastic idea, I will definetily check this out.
Optional parameters is something that I use quite often and yes they are handy as well.
November 15, 2013 at 01:52 am - Permalink