
How to do integration over an internal variable in fitfunc?
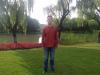
Yuan Fang
Mon, 10/30/2023 - 11:35 pm
I have a fitfunc like this:
function PINEMnewfit(w,x): FitFunc
wave w
variable x
//w[0] : coupling
//w[1] : multii
//w[2]:thetaE
//w[3]:thetaL
//w[4]:thetae
//w[5]:chirp
//w[6]:time0
//w[7]: time
variable i,j,y=0
for(i=-7;i<8;i++)
for(j=-6;j<7;j+=0.1)
y=y+w[1]/pi/w[2]/w[4]*exp(-((w[7]-w[6])-j+w[5]*(x-2.40747*i))^2/w[4]^2)*(besselj(abs(i),abs(2*w[0]*exp(-j^2/w[3]^2))))^2*exp(-(x-2.40747*i)^2/w[2]^2)
endfor
endfor
return y
End
wave w
variable x
//w[0] : coupling
//w[1] : multii
//w[2]:thetaE
//w[3]:thetaL
//w[4]:thetae
//w[5]:chirp
//w[6]:time0
//w[7]: time
variable i,j,y=0
for(i=-7;i<8;i++)
for(j=-6;j<7;j+=0.1)
y=y+w[1]/pi/w[2]/w[4]*exp(-((w[7]-w[6])-j+w[5]*(x-2.40747*i))^2/w[4]^2)*(besselj(abs(i),abs(2*w[0]*exp(-j^2/w[3]^2))))^2*exp(-(x-2.40747*i)^2/w[2]^2)
endfor
endfor
return y
End
Actually I want to do integration from -inf to inf for variable j insted of summing it from -6 to 6. How can I do this?
Or if I want to do integration for j from 0 to a definite value how can I make it?
Thank you!
Integrate1D is used to perform definite integration of a function.
DisplayHelpTopic "Integrate1D"
October 31, 2023 at 03:39 am - Permalink
Are you integrating with respect to the independent variable? If so, you will also want to use an all-at-once fitting function:
DisplayHelpTopic "All-At-Once Fitting Functions"
October 31, 2023 at 12:55 pm - Permalink
Thank you. I successfully write the fitting code using Integrate1D but how to determine integration range? What I want to do is integrating from -inf to inf. But when I take large values as integration boundary it is very slow. Codes are attached below:
wave w
variable x
//w[0] : coupling
//w[1] : multii
//w[2]:thetaE
//w[3]:thetaL
//w[4]:thetae
//w[5]:chirp
//w[6]:time0
//w[7]: time
variable/g a=w[0],b=w[1],c=w[2],d=w[3],f=w[4],g=w[5],h=w[6],k=w[7]
variable ii,y=0
for(ii=-7;ii<8;ii++)
y=y+kernel1(ii,x)
endfor
return y
End
function kernel0(inj)
variable inj
NVAR a,b,c,d,f,g,h,k
NVAR ini,inx
return b/pi/c/f*exp(-((k-h)-inj+g*(inx-2.40747*ini))^2/f^2)*(besselj(abs(ini),abs(2*a*exp(-inj^2/d^2))))^2*exp(-(inx-2.40747*ini)^2/c^2)
end
function kernel1(ii,xx)
variable ii,xx
variable/g ini,inx
ini=ii
inx=xx
return Integrate1D(kernel0,-10,10,1)
end
October 31, 2023 at 08:32 pm - Permalink
I have compared the results of integrating from -10 to 10 and from -20 to 20, they are basically the same. So I determine to use the range -10 to 10. I still want to know is there any way to speed up the code?
October 31, 2023 at 11:34 pm - Permalink
Possibly the best strategy is to integrate from 0 to <largish positive number> and from 0 to <largish negative number>. Be sure the options are correct to get the adaptive integration. Presumably your integrand is large near zero and approaches zero at +-<largish number>. By starting at zero and working outward, the adaptive integration will not spend time trying to add up very small numbers that later turn out to be useless.
Also- if you use the optional parameters, you can pass a coefficient wave to the integrand function rather than messing with global variables. The optional coefficient wave is a relatively new feature.
November 1, 2023 at 09:31 am - Permalink
In reply to Possibly the best strategy… by johnweeks
Thank you!
November 1, 2023 at 05:47 pm - Permalink