
How to create a repeating pattern of a defined time on a graph?
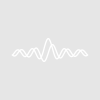
todd22
Sat, 03/05/2011 - 10:52 pm
I am doing electrophysiological experiments in which a repetitive stimulus is applied over a defined time window within a larger sampling period; specifically:
A single sweep is 5 seconds long. Within that 5 seconds, a repetitive stimulus is applied from t=3 to t=4 seconds. The stimulus is pulsed light at 10 Hz, where each light pulse lasts 20ms. What I would like to do is to have each of the light stimuli graphically represented in a temporally accurate way... in other words, to have lines whose x values equal 20ms, and which start at the time points when they were actually applied (i.e. 3.0, 3.1, 3.2, etc), and to be able to include these lines in a graph where the actual recording data is shown.
I've been doing this manually by making separate lines for each stimulus and aligning them... but it is very laborious and I'm guessing there must be an automated way to do this... any advice would be very much appreciated!!
Thank you,
Todd
// Example:
// MakeStimulusPattern(3, 4, 10, .02, 1, "MyStimulus")
// Display MyStimulus_y vs MyStimulus_x
Function MakeStimulusPattern(startTime, endTime, frequency, onTime, pulseLevel, patternName)
Variable startTime // e.g., 3 for 3 seconds
Variable endTime // e.g., 4 for 4 seconds
Variable frequency // e.g., 10 for 10 Hz
Variable onTime // e.g., .02 for 20 ms
Variable pulseLevel // e.g., 1 for lines at Y=1
String patternName // e.g., "MyStimulus"
Variable totalTime = endTime - startTime
Variable numPulses = totalTime * frequency
Variable timeBetweenPulses = 1 / frequency
// We need end points for each pulse and a blank in between pulses
Variable numPoints = 3*numPulses
String xName = patternName + "_x"
String yName = patternName + "_y"
Make/O/N=(numPoints) $xName, $yName
Wave xw=$xName, yw=$yName // Create wave references
Variable i
for(i=0; i<numPulses; i+=1)
xw[i*3] = startTime + i*timeBetweenPulses
xw[i*3+1] = xw[i*3] + onTime
xw[i*3+2] = NaN // Blank to separate pulses
yw[i*3] = pulseLevel
yw[i*3+1] = pulseLevel
yw[i*3+2] = NaN // Blank to separate pulses
endfor
End
Paste into the procedure window and execute the example commands shown in the comments.
March 6, 2011 at 05:35 am - Permalink
->Windows -> Procedure window -> Procedure Window, and then pasted your text into the window. But after that I got stuck.. am I supposed to 'execute' that procedure while a graph is open? If so, how do I do that? Also, is this going to draw lines of defined width and placement in the graph which I can modify after they are drawn (change color, line thickness, etc..)?
Sorry if these are mundane questions but I have not used procedures before...
Thank you again for your help -
Todd
March 6, 2011 at 04:03 pm - Permalink
Display MyStimulus_y vs MyStimulus_x
The first line calls ("executes") Howard's function, which just calculates a new pair of data waves called MyStimulus_x and MyStimulus_y (since you have called the function with the argument "MyStimulus").
The second line displays the data in a new graph window. You could have the same result by choosing "New Graph" form the Window menu and selecting these waves manually. Naturally, you have all the ways to adapt the style of the stimulus trace as you have for your experimental data.
If you want to plot the stimulus wave in the same graph as your experimental data, you can either use the menus or execute the following line from the command window (your experimental graph being in front):
AppendToGraph MyStimulus_y vs MyStimulus_x
For further work with the procedure window and programming, it would be best if you consulted the manual or did the tutorial...
March 6, 2011 at 04:45 pm - Permalink
Please do the first half of the Igor Guided Tour. You can find it by choosing Help->Getting Started or in Volume I of the Igor PDF manual.
Doing the Guided Tour, or at least the first half of it, is essential for using Igor.
March 6, 2011 at 05:06 pm - Permalink
I took a look at the procedure and tried to make a few changes (e.g. changing the Variable timeBetweenPulses = 1 / frequency to 1000/frequency) but did not get the desired output.. could you tell me what needs to be amended?
March 6, 2011 at 11:11 pm - Permalink
// Example:
// MakeStimulusPattern(3000, 4000, 10, 20, 1, "MyStimulus")
// Display MyStimulus_y vs MyStimulus_x
Function MakeStimulusPattern(startTime, endTime, frequency, onTime, pulseLevel, patternName)
Variable startTime // e.g., 3000 for 3000 milliseconds
Variable endTime // e.g., 4000 for 4000 milliseconds
Variable frequency // e.g., 10 for 10 Hz
Variable onTime // e.g., 20 for 20 ms
Variable pulseLevel // e.g., 1 for lines at Y=1
String patternName // e.g., "MyStimulus"
Variable totalTime = endTime - startTime
Variable millisecondsBetweenPulses = 1000 / frequency
Variable numPulses = totalTime / millisecondsBetweenPulses
// We need end points for each pulse and a blank in between pulses
Variable numPoints = 3*numPulses
String xName = patternName + "_x"
String yName = patternName + "_y"
Make/O/N=(numPoints) $xName, $yName
Wave xw=$xName, yw=$yName // Create wave references
Variable i
for(i=0; i<numPulses; i+=1)
xw[i*3] = startTime + i*millisecondsBetweenPulses
xw[i*3+1] = xw[i*3] + onTime
xw[i*3+2] = NaN // Blank to separate pulses
yw[i*3] = pulseLevel
yw[i*3+1] = pulseLevel
yw[i*3+2] = NaN // Blank to separate pulses
endfor
End
March 7, 2011 at 06:29 am - Permalink
March 8, 2011 at 01:43 am - Permalink