
Getting the data folder of a wave in the top graph.
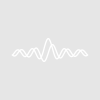
reepingk
Thu, 05/05/2016 - 09:09 am
Function iMaxCalc()
String list = TraceNameList( "", ";", 1), maxWave //gets waves in top graph
Variable numItems = ItemsInList( list ), i, y_Max, y_Min, lengthh, x_Max //variables
for(i=0;i<numItems;i+=1)
maxWave = stringfromlist(i,list,";") //gets the first listed wave
print maxWave //prints the name for reference
Wave workingWaveY = $maxWave //should reference workingWaveY to the name of the wave gotten in the first part.. doesn't work... probably due to being in the wrong data folder
Print GetWavesDataFolder(workingWaveY,1) //doesn't work because the above didn't work
endfor
end
String list = TraceNameList( "", ";", 1), maxWave //gets waves in top graph
Variable numItems = ItemsInList( list ), i, y_Max, y_Min, lengthh, x_Max //variables
for(i=0;i<numItems;i+=1)
maxWave = stringfromlist(i,list,";") //gets the first listed wave
print maxWave //prints the name for reference
Wave workingWaveY = $maxWave //should reference workingWaveY to the name of the wave gotten in the first part.. doesn't work... probably due to being in the wrong data folder
Print GetWavesDataFolder(workingWaveY,1) //doesn't work because the above didn't work
endfor
end
How am I supposed to get the data folder for the wave before being able to make a wave reference using the wave's name?
In fact, the "Wave" statement doesn't work even if I manually set it to the right data folder... (It does work if I manually type in the data folder's path into the procedure, but then it's a chicken and the egg type problem, see below.)
Wave workingWaveY = $("root:LSVCompilationTrimmed:'040815_650CH3Cl_CH4_Cl':" + maxWave)
Works, but doesn't help me.
(Yes, I know a different command is recommended than GetWavesDataFolder, but I just wanted to see if it knew where it was, and this command returned the full path, wasn't sure if a data folder reference would print or not...)
The full path for the first wave in the list should be this ... root:LSVCompilationTrimmed:'040815_650CH3Cl_CH4_Cl':'1606Methane2.0_0pmaLSV2_0'
Variable index = 0
do
WAVE/Z workingWaveY = WaveRefIndexed("", index, 1)
if (!WaveExists(workingWaveY))
break
endif
index += 1
while (1)
end
Alternately, you could use TraceNameList to get a list of trace names and then use TraceNameToWaveRef to get a wave reference to the corresponding Y wave for each trace.
May 5, 2016 at 10:38 am - Permalink
Ah, traceNametoWaveRef works well. That's the magic keyword I was looking for that did what I wanted. Works super well, thanks.
May 5, 2016 at 11:52 am - Permalink
I wasn't sure how to do degree minute second math in igor, so I just did it manually by multiplying and dividing by 60 when necessary.
Menu "Macros"
"iMaxCalc", iMaxCalc()
End
//Macro iMaxCalc()
// iMaxCalc()
//End
Function iMaxCalc()
String list = TraceNameList( "", ";", 1), maxWave, folderName
Variable numItems = ItemsInList( list ), i, y_Max, y_Min, lengthh, x_Max, iMax, slope,timeHours,timeMins,timeExtra,origTimeHours=14,origTimeMins=16
Prompt origTimeHours, "Enter Hours"
Prompt origTimeMins, "Enter Mins"
DoPrompt "Enter Time", origTimeHours, origTimeMins
for(i=0;i<numItems;i+=1)
maxWave = stringfromlist(i,list)
lengthh = strlen(maxWave) -3
Wave workingWaveY = tracenametowaveref("", maxWave)
y_Max = WaveMax(workingWaveY)
y_Min = WaveMin(workingWaveY)
maxWave = maxWave[0,lengthh] + "1'"
// print maxWave
// folderName = GetWavesDataFolder(workingWaveY,1)
Wave workingWaveX = $(GetWavesDataFolder(workingWaveY,1) + maxWave)
x_Max = WaveMax(workingWaveX)
slope = (y_Max-y_Min)/(0-x_Max)
iMax = ((-y_Max)/slope)
slope = slope*1000
timeHours = str2num(maxWave[1,2])
timeMins = str2num(maxWave[3,4])
timeExtra = timeHours + (timeMins /60)
timeExtra = (timeExtra - (origTimeHours + (origTimeMins/60)))*60
Print "Time: " + maxWave[1,4]
Print "Cl Time: " + num2str(timeExtra)
Print "iMax: " + num2str(iMax)
Print "Slope: " + num2str(slope)
Print "**************"
endfor
End
May 5, 2016 at 12:34 pm - Permalink