
Getting around for loops in image processing
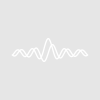
deconite
Fri, 05/16/2014 - 10:46 am
For matrices there exist scalar functions that apply automatically to each index in a pair (or more) of matrices. It's easy to take a cosine of a whole matrix, ect. Does there exist a way to apply if-then actions to each index of a wave individually without constructing a for-loop that runs through the indicies of the wave? I imagine that'd save some time if igor didn't have to re-interprete the if-statement every time it looked at a different scalar in a wave.
Look on page 173 of the manual and for Operators in IV-5.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
May 16, 2014 at 11:57 am - Permalink
Yes. Here is a demo of what you can do:
// Setup()
// mat = NaN
// Demo1()
// mat = NaN
// Demo2()
// mat = NaN
// Demo3()
Function Setup()
Wave/Z mat
if (!WaveExists(mat))
Make/N=(5,5) mat = NaN
Edit mat
endif
End
Function Demo1()
Wave mat
mat = p==q ? 1:0
End
Function Demo2()
Wave mat
mat = SelectNumber(p==q, 0, 1)
End
Function Helper3(pp, qq)
Variable pp, qq
if (pp == qq)
return 1
endif
return 0
End
Function Demo3()
Wave mat
mat = Helper3(p, q)
End
For details execute:
DisplayHelpTopic "Waveform Arithmetic and Assignment"
The whole topic will be a good refresher. The section "Example: Comparison Operators and Wave Synthesis" addresses your question.
May 16, 2014 at 12:01 pm - Permalink
May 16, 2014 at 12:29 pm - Permalink