
Easy way to mask waves?
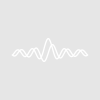
amellnik
Mon, 01/02/2012 - 11:41 am
targetwave = targetwave/(maskwave==0)
WaveTransform zapINFs targetwave
WaveTransform zapNaNs targetwave // 0/0 = NaN
This has the obvious problem that it gets rid of any NaNs and INFs that should be in targetwave. What's the proper way to do this? Thanks
-Alex
How about something like this?
Wave w //wave to have points deleted
Wave wMask //comparison wave; zeroes here will delete corresponding points in w
//w and wMask are assumed to be same length
Variable vNumPoints
Variable index
vNumPoints = DimSize(w,0)
//have to do this from last point to first otherwise deleting points will change index of all points forward
for(index = vNumPoints - 1; index >= 0; index -= 1)
if(wMask[index] == 0)
DeletePoints index, 1, w
endif
endfor
End
My quick test shows that any point in wmask with a value of zero will cause the corresponding point in w to be deleted. There is no error checking, so watch out if it behaves unexpectedly. Hope this is what you have in mind.
January 2, 2012 at 03:19 pm - Permalink
Extract/O targetwave, targetwave, maskwave!=0
should also work. Be careful, targetwave is being overwritten! To avoid this, use a new name for destwave.A
January 8, 2012 at 12:48 am - Permalink
copyWave=sourceWave[pcsr(A):pcsr(B)]
however that does not work. What would be the proper code to do this?
January 9, 2012 at 02:27 am - Permalink
January 9, 2012 at 07:11 am - Permalink
January 16, 2012 at 05:16 am - Permalink
May 17, 2012 at 11:37 am - Permalink