
Calculating distance from longitude and latitude points
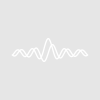
LaMP
Wed, 01/22/2014 - 01:54 am
I have three waves: latitude, longitude, and altitude.
I would like to know if there is IGOR code that would allow me to calculate the distance between each latitude and longitude point.
Thanks very much
January 22, 2014 at 03:21 am - Permalink
http://en.wikipedia.org/wiki/Haversine_formula
does not address the altitude component, however.
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
January 22, 2014 at 08:48 am - Permalink
January 22, 2014 at 05:13 pm - Permalink
You're right, of course. I was just pointing out what the algorithm was, and that it was for a constant altitude.
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
January 22, 2014 at 06:20 pm - Permalink
A.
January 22, 2014 at 11:06 pm - Permalink
The Wikipedia article on Haverside does recognise this and provides a reference to Vincenty's formula which treats Earth as an oblate spheroid.
http://en.wikipedia.org/wiki/Vincenty%27s_formulae
This is an iterative algorithm, so somewhat more complicated to implement than the Haverside formula. Which is appropriate depends upon how accurate one needs the answer to be.
K
January 22, 2014 at 11:25 pm - Permalink
I needed to do this in Igor today and found this thread. The replies identified the answer but there was no solution posted. So , here is a quick function to do it:
// uses haversine formula
// radius of earth is taken to be 6375 km (approximate for London, UK)
Function DistanceBetweenTwoPoints(x0,y0,x1,y1)
Variable x0,x1,y0,y1
Variable dlon = pi * ((x1 - x0) / 180)
Variable dlat = pi * ((y1 - y0) / 180)
Variable aa = (sin(dlat / 2)) ^ 2 + cos(pi * (y0 / 180)) * cos(pi * (y1 / 180)) * (sin(dlon / 2)) ^ 2
Variable cc = 2 * atan2(sqrt(aa), sqrt(1 - aa))
Variable dd = 6375 * cc
// return distance in metres
return dd * 1000
end
// example usage with two 1D waves called lat and lon, distance between rows 0 and 1
Print DistanceBetweenTwoPoints(lon[0],lat[0],lon[1],lat[1])
February 23, 2020 at 08:29 am - Permalink