
Basic Q- How can I stitch together multiple images and how do I append a variable to a string?
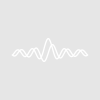
jrysocas
Fri, 07/12/2013 - 02:12 pm
Function CreateSections()
variable i=0
variable j=10
do
duplicate/o/r=((j-10),j)(0,10) fly section_i
newimage section_i
AutoPositionWindow/E $WinName(0,1)
j=j+10
i=i+1
while(i<=10)
End
variable i=0
variable j=10
do
duplicate/o/r=((j-10),j)(0,10) fly section_i
newimage section_i
AutoPositionWindow/E $WinName(0,1)
j=j+10
i=i+1
while(i<=10)
End
Ideally, completion of this program will yield 11 images named section_0, section_1, section_2, ..., and section_10. Instead, only one image called section_i is created. What is the appropriate syntax for appending a variable to a string? Also, what function could I use to stitch these images back together after the program runs to completion?
Thanks in advance,
-jry
Your code segment for creating the sub-images should be changed. If I understand correctly that you want 10x10 images out of 100x100 image you would want to use for row rr and column cc:
String imageName="wave_"+num2str(rr)+"_"+num2str(cc)
Variable rs=rr*10
Variable cs=cc*10
// copy the data into a new wave:
Duplicate/O/R=[rs,rs+9][cc,cc+9] fullImageWave,$imageName
I hope this helps,
A.G.
WaveMetrics, Inc.
July 12, 2013 at 05:08 pm - Permalink
Use + to append strings. Use num2istr or num2str to convert a number to a string. You could also use sprintf.
Here is a solution for your task. I separated the step of creating the sub-images from the step of creating the plots. This splits the function into two clear parts which makes each of them easier to code, read and use.
String baseName
Variable startRow, startColumn
String name = "SubImage_" + num2istr(startRow) + "_" + num2istr(startColumn)
return name
End
Function CreateSubImages(wIn, baseName, startColumn, endColumn)
Wave wIn // Assumed to be a 100x100 matrix
String baseName // Base name for output waves - e.g., "SubImage"
Variable startColumn, endColumn // e.g., 0, 9 for the first 10 columns
Variable startRow=0, endRow
for(startRow=0; startRow<100; startRow+=10)
endRow = startRow + 9
String name = GetSubImageName(baseName, startRow, endRow)
Duplicate /O /R=[startRow,endRow][startColumn,endColumn] wIn, $name
Wave subImageWave = $name // You would use this if you wanted to do something with the wave just created by Duplicate
endfor
End
Function DisplaySubImages(baseName, startColumn, endColumn)
String baseName
Variable startColumn, endColumn // e.g., 0, 9 for the first 10 columns
Variable startRow=0, endRow
for(startRow=0; startRow<100; startRow+=10)
endRow = startRow + 9
String name = GetSubImageName(baseName, startRow, endRow)
Wave subImageWave = $name
NewImage /K=1 subImageWave // /K=1 means "kill with no dialog" if user closes graph window
String graphName = WinName(0,1)
AutoPositionWindow/E $graphName
endfor
End
Function Demo()
Make/O/N=(100,100) mainImage = p + 10*q
String baseName = "SubImage"
Variable startColumn=0, endColumn=9 // First 10 columns - 0 through 9
CreateSubImages(mainImage, baseName, startColumn, endColumn)
DisplaySubImages(baseName, startColumn, endColumn)
End
July 12, 2013 at 05:24 pm - Permalink